Debugging JavaScript Errors
Failed to fetch
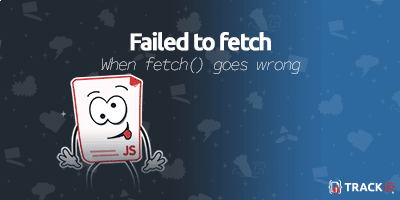
Network errors happen, and the Fetch API has certain ways of telling you that. One of them is the uninspiring error message Failed to fetch
.
A certain amount of random network gremlins are to be expected on the internet, but this error can indicate a real problem with your web application.
Keep in mind: Browsers return different messages for this error!
Each major browser family uses very different text for this kind of fetch error. Chrome’s Failed to fetch
variation is very common because Chromium based browsers are widely used.
-
Chrome/Chromium/Microsoft Edge
Failed to fetch
-
Safari
Load failed
-
Firefox
NetworkError when attempting to fetch resource.
Understanding the error
This error is thrown by the Fetch API when the browser fails to get any response from the requested URL. The error will NOT occur if the server returns data, even a failed response.
Something in your code is calling fetch and not getting a reply from the server. That is, a call like this is being made:
// fetch with promises
// editorial comment: I do NOT miss promises!
fetch("https://api.example.com:3000/broken/endpoint")
.then((response) => response.json()
.then((json) => {
/* do something with the response! */
console.log(json)
})
)
// fetch using async
var response = await fetch("https://api.example.com:3000/broken/endpoint")
var json = await response.json()
console.log(json)
Browsers
- Chrome/Microsoft Edge/Chromium:
Failed to fetch
- Firefox:
NetworkError when attempting to fetch resource.
- Safari:
Load failed
Finding the root cause
There are a few common reasons for requests to receive no response from the server. Some more common than others.
Misconfigured CORS headers are a common cause of this problem. The browser immediately aborts a cross-domain request when it detects that the server has not returned the proper CORS headers.
Secondly, ad blockers are a very common cause of Fetch errors. They’ve become a fact of life for a large percentage of browsers on the internet. Any requests matching the ad blocker’s (very broad) rules are aborted before they are sent.
Page navigation cause this issue as well. The browser aborts all outstanding fetch requests when the user navigates to a new page.
Lastly, the server was simply not reachable. A service that is down, misconfigured or even a misspelled domain name can be at fault. Anything where the server is entirely unreachable.
Can I ignore this error?
failed to fetch
can indicate a real problem in your web application. You’ll need to identify WHY fetch requests were aborted before ignoring this error. A server configuration or application bug may be to blame. In other cases, the error can be ignored when they are caused by things outside your control like ad blockers or page navigations.
Handling the error will prevent it from appearing in the console or any error monitoring services like TrackJS. Trapping the error differs depending on whether async or the promise API is being used:
fetch("https://api.example.com:3000/broken/endpoint")
.then((response) => response.json()
.then((json) => {
/* do something with the response! */
console.log(json)
})
)
.catch((error) => {
console.log("Fetch failed, but we caught the error. Error: ", error.message)
})
var response = null;
try {
response = await fetch("https://api.example.com:3000/broken/endpoint")
}
catch(error) {
console.log("Fetch failed, but we caught the error. Error: ", error.message)
}
var json = await response.json()
console.log(json)
How to fix
Fixing this error depends on its cause. Four causes are usually to blame:
1. CORS Errors
Cross-Origin Resource Sharing (CORS) errors occur when the page fetches data from a different domain than the one the page lives on (eg - a page on example.com makes a request to api.example.com). The error is usually the result of a misconfigured service or application. The API server must return CORS headers indicating the page has permission to make the request.
A good place to start is to ensure the server is returning the correct Access-Control-Allow-Origin
header in the response. Your situation may be more complex, as CORS can be configured a number of ways.
2. Ad Blockers
Sometimes ad blockers are just a fact of life. If you have enough control over the fetch call, you can avoid ad blockers.
Forwarding domains prevent third party APIs from being blocked. Forwarding domains use a first party domain to forward all requests to the actual service (eg - my-api.example.com
would forward to api.the-actual-service.com
). This is the method we use on our products. TrackJS supports configuring a custom forwarding domain to prevent ad blockers from stopping error monitoring data.
3. Page Navigation Aborting Fetch
Fetch cancellations caused by page navigation are usually sporadic and can be ignored.
If the errors are occurring frequently, it could indicate very slow fetch calls. Slow services make it more likely for the call to be outstanding when the user navigates away from the page. Slow services may also impact page rendering, causing the user to navigate away in frustration.
4. Server or Service Not Responding
Failed connections or empty responses usually indicate that a server or service is down. Further investigating into that service will be needed. Messages like ERR_CONNECTION_REFUSED
or ERR_EMPTY_RESPONSE
appear in the Chrome developer tools in these cases.
Connections also fail when the request URL’s domain is invalid or cannot be resolved. Chrome will place an additional message in the developer console stating, ERR_NAME_NOT_RESOLVED
.
Monitor Your Environment!
Did your changes fix the issue? Is the issue fixed in production? How do you know? Errors come from lots of different places on the web. Some are noise, but many will cause break your page, upsetting users. TrackJS can tell you when errors happen, and more importantly, how to fix them.