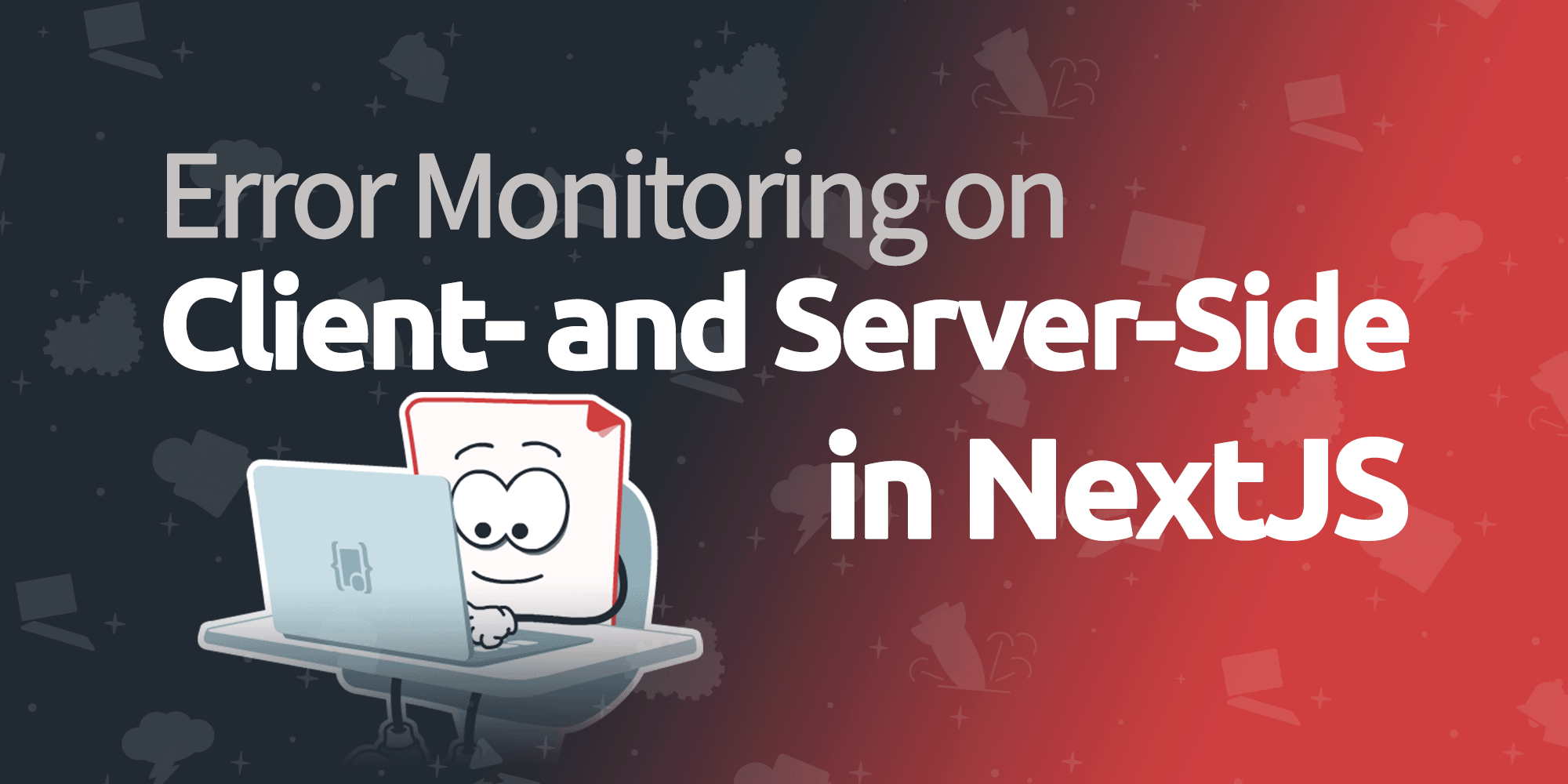
Error Monitoring on Client- and Server-Side in NextJS 14+
NextJS is the hot JavaScript framework right now, and like all JavaScript, it can cause quite a few bugs on both the client- and server-side of your applications.
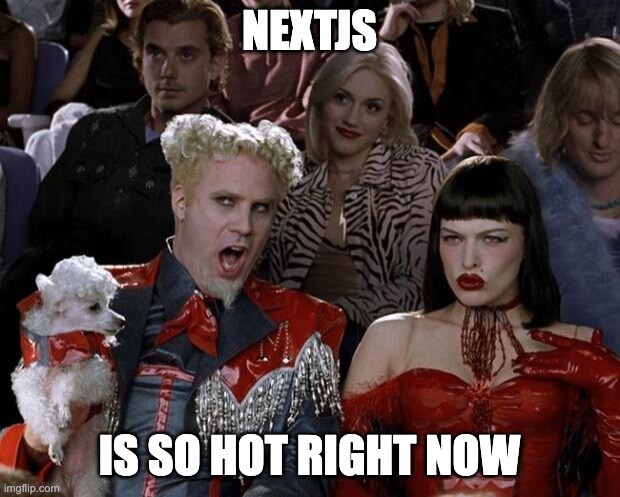
One of the most powerful features of NextJS is enabling you to use your code, templates, and patterns across both the server and the client. NextJS will mostly figure out the most efficient place to run. This is super powerful and makes NextJS applications feel very fast compared to strictly client-side rendered applications.
However, that does mean you need to handle errors and exceptions in both the client-side browser environment as well as the NodeJS server. There are two slightly different ways to make sure you monitor all the ways your NextJS application can fail.
Client-Side Error Handling in NextJS
NextJS is built on top of React and utilizes the ErrorBoundary concept to handle errors at different levels of the application. This could be useful if you want to have different error screens for different parts of the app.
Of course, we can’t predict where errors will occur, so we also need a “top level” error handler to catch everything. This is also the best place to plug in an error monitoring service like TrackJS. In NextJS, the top-level error handler is the global-error.tsx
file in the root of app
.
"use client";
import NextError from "next/error";
import { useEffect } from "react";
import { TrackJS } from "trackjs";
// Setup your error monitoring
TrackJS.install({...});
export default function GlobalError({ error, reset }) {
useEffect(() => {
// Capture an error
TrackJS.track(error);
}, [error]);
return (
<html>
<body>
{/* This is the default Next.js error component. */}
<NextError statusCode={undefined as any} />
</body>
</html>
);
}
The global-error.tsx
file only runs in the client-side environment, so it needs to start with the NextJS "use client"
directive.
Quick aside, the TrackJS agent has been using the custom "use awesome"
directive for over 10 years now. Because, ya know, it’s awesome.
Most of this global-error.tsx
is the standard boilerplate, with 2 additions: first, the call to TrackJS.install
. This is where you can configure your instrumentation of the client-side to gather any additional context for your errors. This code will be run right away, regardless of whether or not an error occurs.
The other addition is the call to TrackJS.track
within the useEffect
. This get’s called by NextJS when an error actually has occurred in a production environment. Even if your error monitoring automatically captures errors (like TrackJS), sending the errors directly within the useEffect
will provide better error data.
Don’t Forget! The global-error.tsx
only runs in production builds. To test out your error handling, you need to build and start the project in production mode.
Server-Side Error Handling in NextJS
Some of your application code will also be run on the NodeJS server-side of NextJS. NextJS does a pretty good job of detecting most failures, and when it does, it routes the errors to the client-side where it will be recorded by the global-error.tsx
file.
But it doesn’t catch everything.
There are several situations where NextJS can blow up on the server and not return anything to the client. How can you detect when those errors happen?
There is no app.tsx
or other root application file to plug into. However, the root layout.tsx
file is one of the first things that runs on both client and server, so it’s a great place to add other application-level configuration and calls.
import type { Metadata } from "next";
import { TrackJS } from "trackjs-node";
// Setup your error monitoring
TrackJS.install({...});
export const metadata: Metadata = {...};
export default function RootLayout({ children }: Readonly) {
return (/*your layout markup*/);
}
Your layout.tsx
file will look much more complicated as it will have lots of general structure and layout for your application. But where I have the call to TrackJS.install
is a great place to set up general error handling that will run on the server. An error monitoring system like TrackJS will attach itself to all the places a NodeJS application can fail and record errors automatically.
Note On the server-side, we use a trackjs-node
package instead of the standard browser agent. You probably want to implement an isomorphic wrapper around both agents to centralize your configuration, which is very easy to do.
Build NextJS Apps with Confidence!
Bugs are inevitable, even in modern frameworks. Having a system to automatically capture and report errors when they happen is key to having confidence in your development cycle, and your products.
TrackJS error monitoring fully supports NextJS, and all other JavaScript technologies. Built by developers for developers.