JavaScript error monitoring
Catch bugs before users do
TrackJS automatically detects JavaScript errors with a story about how the error happened, allowing you to fix critical bugs before your users even notice them. Stop relying on customer reports and start resolving issues.
“With TrackJS, we're empowered to proactively resolve UI errors the moment they occur instead of passively waiting for customers to report issues.”
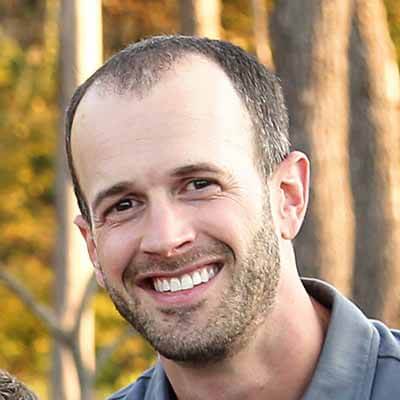
Loved by developers
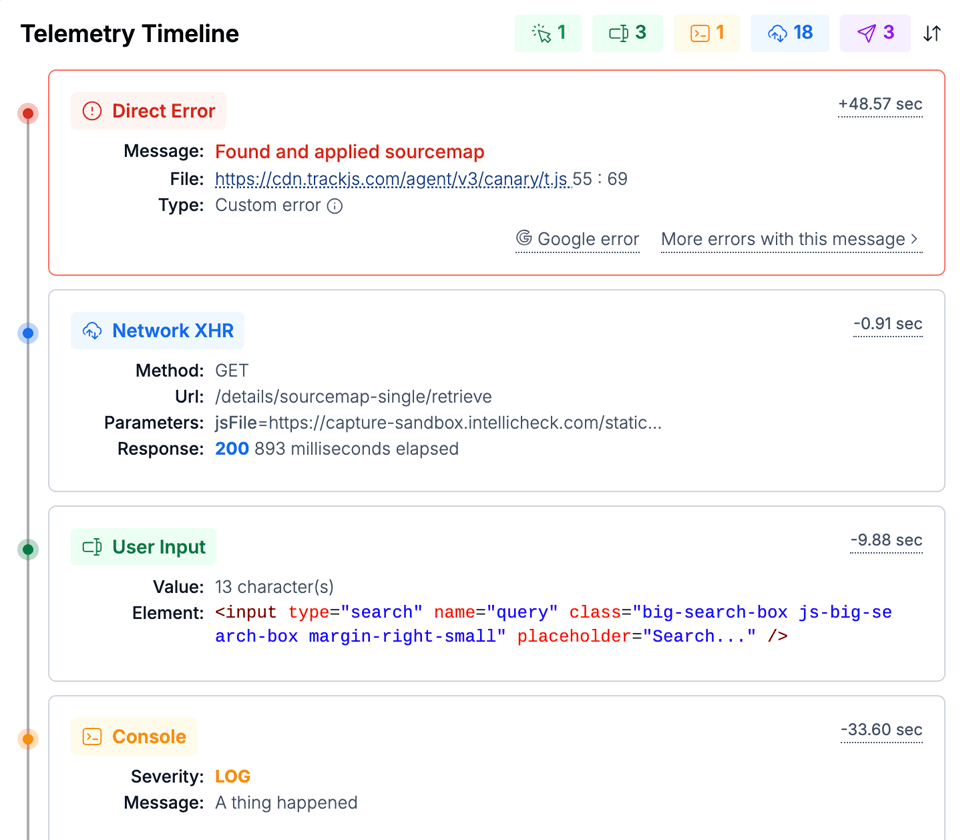
Telemetry Timeline
The full story behind every error
Understand exactly what happened before each error with a chronological timeline of network requests, user interactions, console logs, and navigation events. Quickly reproduce and resolve every issue without the performance overhead and cost of session replay tools.
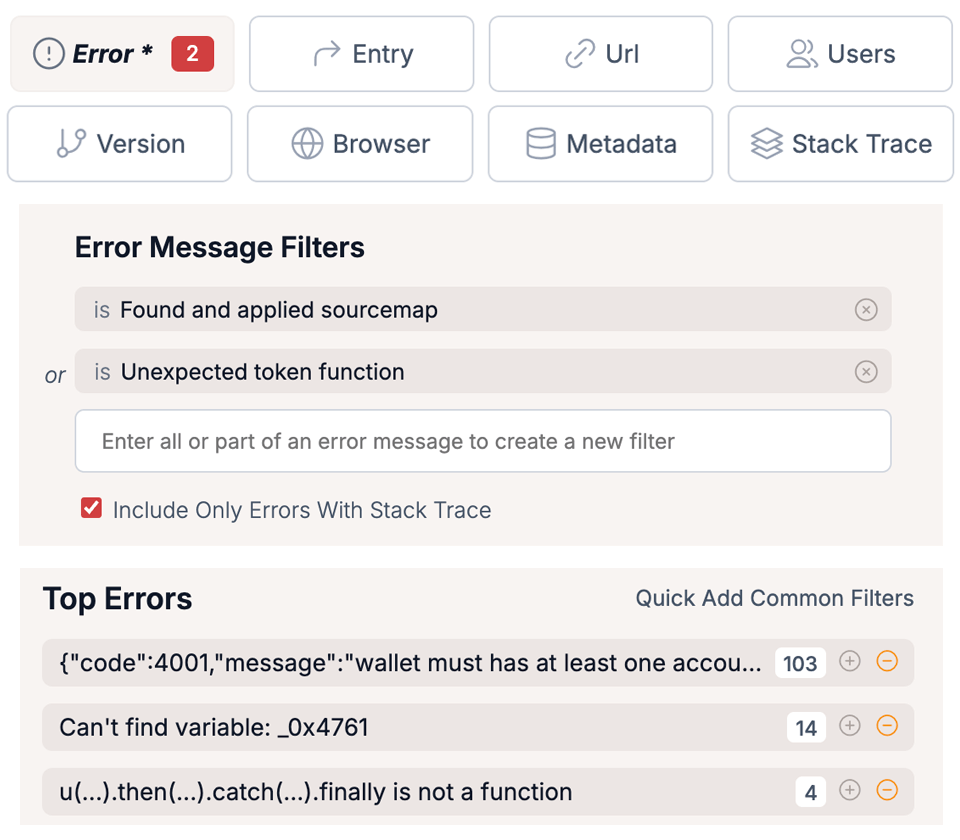
Discoverable Error Filters
Instant error filtering and searching to find issues fast
Zero in on what matters with intuitive filtering tools that require no query language to learn. Quickly identify patterns across users, browsers, and pages with automatically highlighted trends and one-click filtering. Make data-driven decisions about which errors deserve immediate attention without wasting time crafting queries.
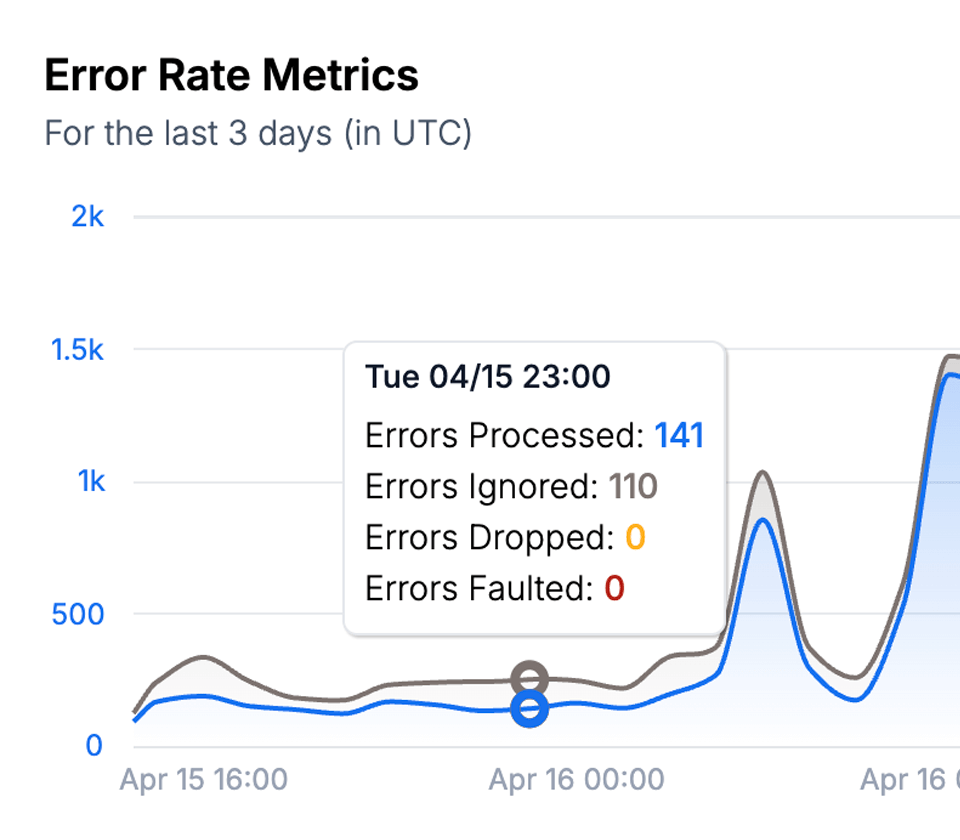
Unlimited Ignore Rules
Focus on the errors that really matter to users
Cut through the noise of third-parties and expected errors with powerful server-side ignore rules, unlimited with every plan. Create custom rules based on any error criteria to automatically filter out known issues, third-party glitches, or low-priority warnings. Keep your dashboard focused exclusively on actionable errors that impact your users.
Installation
Simple integration with your tools
Add TrackJS to your application in minutes, not days. Our lightweight JavaScript error monitoring works seamlessly with all modern frameworks and build tools, requiring minimal configuration to start capturing meaningful error data. Just choose your platform below to get started.
index.html
<script
src="https://cdn.trackjs.com/agent/v3/latest/t.js">
</script>
<script>
TrackJS.install({ token: "your-account-token" });
</script>
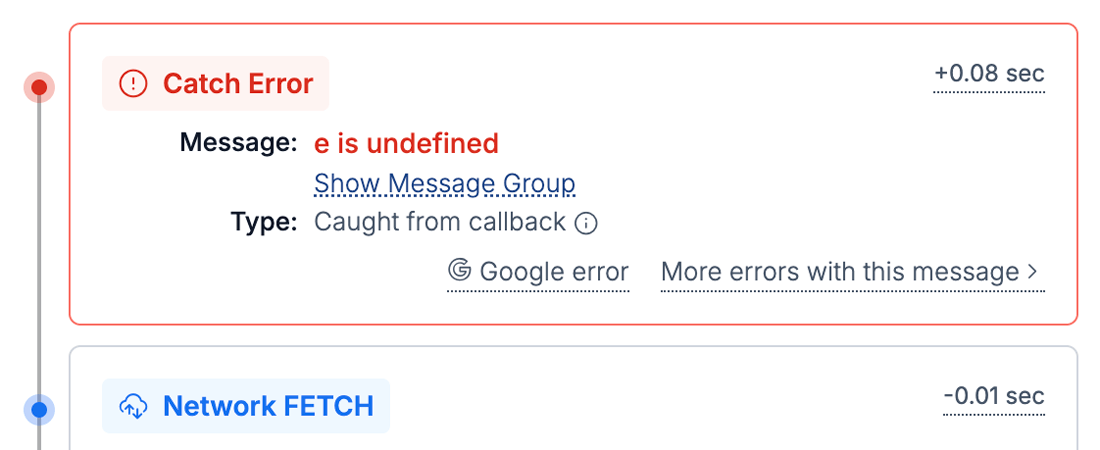
App.jsx
import React, { Component } from "react";
import { TrackJS } from "trackjs";
class TrackJSErrorBoundary extends Component {
componentDidCatch(error, errorInfo) {
TrackJS.track({ error });
this.setState({ error });
}
}
TrackJS.install({ token: "your-account-token" });
const App = () => {
return (
<TrackJSErrorBoundary>
<...>
</TrackJSErrorBoundary>
)
}
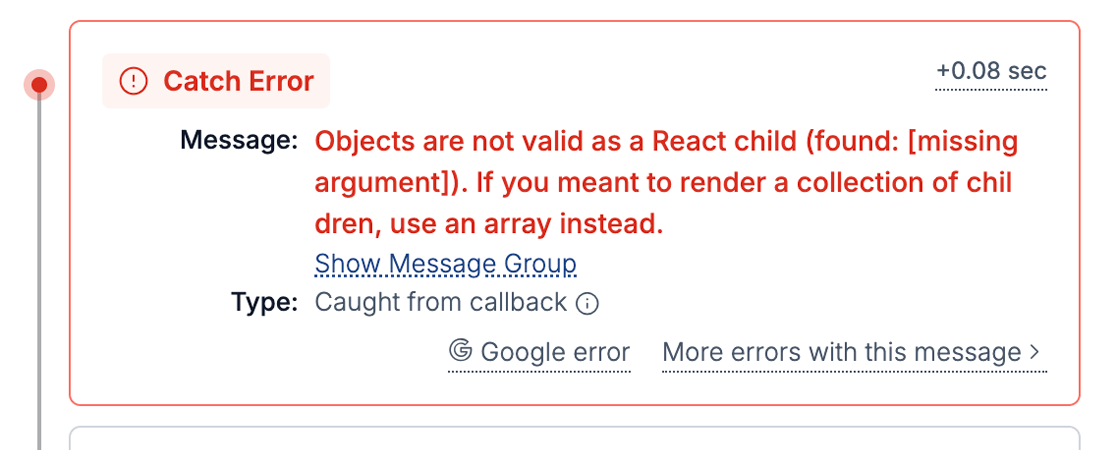
app.module.ts
import { ErrorHandler, Injectable } from "@angular/core";
import { TrackJS } from "trackjs";
import { AppComponent } from "./app.component";
TrackJS.install({ token: "your-account-token" });
@Injectable()
class TrackJSErrorHandler implements ErrorHandler {
handleError(error:any) {
TrackJS.track(error.originalError || error);
}
}
@NgModule({
declations: [AppComponent],
bootstrap: [AppComponent],
providers: [{ provide: ErrorHandler, useClass: TrackJSErrorHandler }]
})
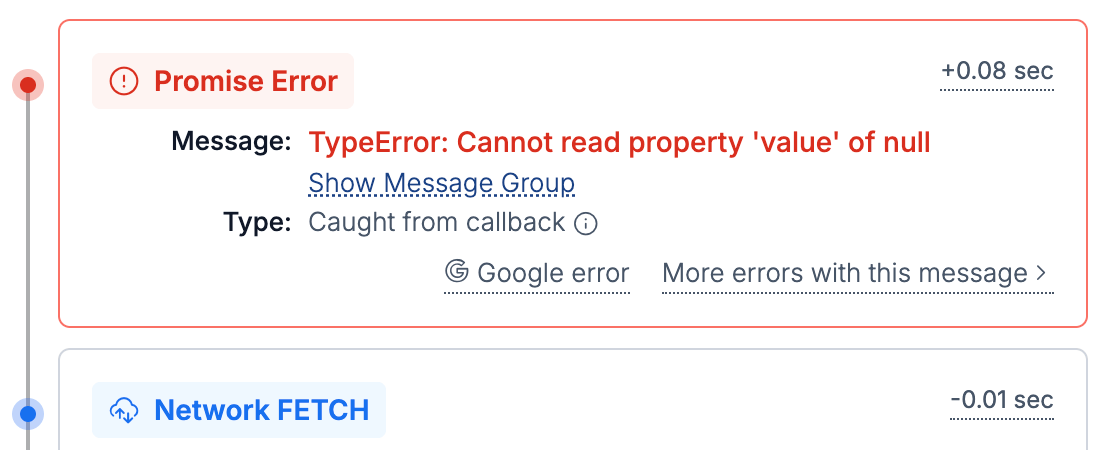
express.js
const express = require("express")
const TrackJS = require("trackjs-node").TrackJS;
TrackJS.install({ token: "your-account-token" });
const app = express()
.use(TrackJS.handlers.expressRequestHandler())
.use(...)
.use(TrackJS.handlers.expressErrorHandler({ next: false }));
.listen(3000);
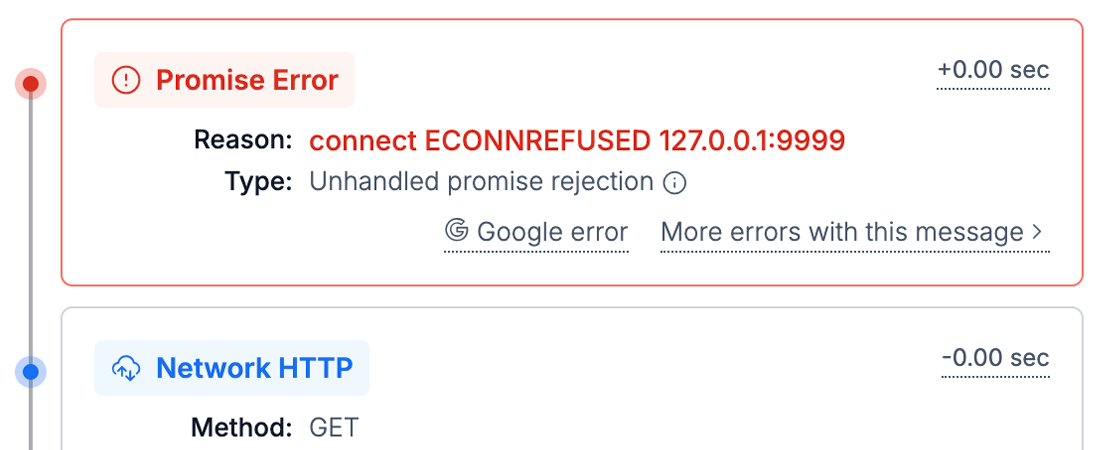
pages/app.tsx
import App from "next/app";
import { TrackJS } from "trackjs-nextjs";
TrackJS.install({ token: "your-account-token" });
export default App;
// NextJS requires a few changes. Check out the docs at
// https://docs.trackjs.com/browser-agent/integrations/nextjs15/
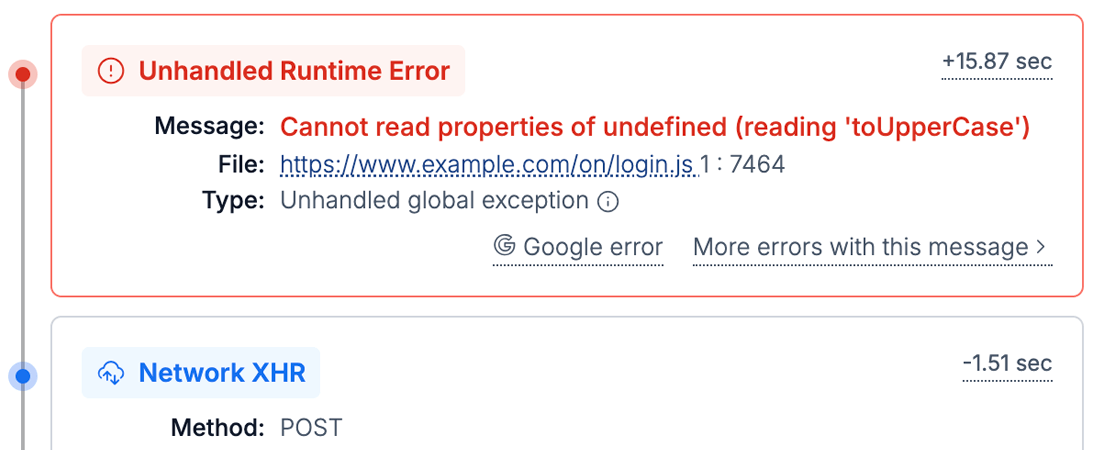
Start your free trial
TrackJS pricing aligns with your success–not your problems. Save thousands of developer hours debugging and grow your business. We've got your back.
Starter
Frequently asked questions
Why are prices based on traffic instead of errors?
Most services price based on errors, which means they profit when you fail. We want to align our success with yours, and grow as you become more successful.
Are there limits on how many errors I can send?
No, you can send us unlimited errors, but we will only keep a limited number of them based on your plan. Error limits are applied every minute, which means you'll always get a steady flow of errors throughout the entire billing period.
You can utilize Ignore Rules, which are included with every plan, to focus TrackJS on the most important errors for your users. Ignored errors are removed before any limit is applied.
Does TrackJS impact my website performance?
Every script added to your page has some incremental cost, TrackJS included. However, the agent is optimized to be less than 8 kb, and self-hostable to reduce connection overhead.
When error data needs to be sent, it's all non-blocking and asynchronous, so it won't slow down the user experience at all.
Does TrackJS capture or store sensitive user data?
No. TrackJS is designed with privacy in mind and does not automatically capture or store sensitive user data. We collect technical information about errors, including stack traces, browser information, and user actions that led to the error—but never passwords, personal information, or sensitive data. Additionally, we provide tools to mask or exclude specific data fields from being collected, and we comply with GDPR, CCPA, and other privacy regulations. Your users' privacy remains protected while you gain the debugging insights you need.
IP Addresses can be captured, truncated, or ignored based on your team's privacy preferences.
Does TrackJS work with minified JavaScript code?
Absolutely, yes! TrackJS automatically pulls the code referenced in error stack trace's, and formats it so you can actually understand what's happening.
If you can produce sourcemaps as part of your JavaScript build, TrackJS can interpret those as well to show you the original commented and formatted source for your errors. Even in other languages like Typescript.